|
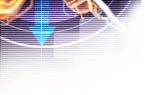 |
JSON File Formats
JSON Can Be Used For 3D Data |
|
|
|
The json file format is a great! Not just for 3d data but for lots of things. It's very popular for web-based systems (storing and transfering data).
The file format itself is text-based and easy to read - but even greater - lots of languages (Java, Python, JavaScript, ..) all have builtin or free packages for parsing or creating json files! So you don't need to do the work of processing each character.
The file format is simple enough - as it's just text.
 | Blender Exporter (Vertices and Faces) |  |
A few cool little things - in blender you can export your data as 'json'. Just open up Blender (3.x), select the object you want to export - select the Scripting tab at the top - and you can write/run small python programs.
The following is a juicy little python script for Blender that exports the mesh data.
|
|