|
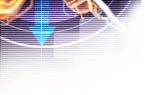 |
Tutorials - Tips - Secrets - for C and C++..
It does all the hard work for us.. |
|
|
|
Optimising Your C
by bkenwright@xbdev.net
 | C/C++ Code Optimization Techniques |  |
Everyone learning C or even C++ should know the basics of optimizing your code. No matter how fast your CPU gets, you always want to squeeze more performance out of it. Let's go through some common optimization techniques that are useful to know and might improve your code.
Lightning Fast Multiplication and Division
When someone first asked me, "How can you improve this line of code?"
|
|