|
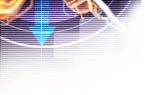 |
JavaScript...
so much power in such a few lines of code.. |
|
|
|
JavaScript is a versatile programming language used primarily for web development;
Breakdown of the basic JavaScript syntax, structure, and layout.
1. Comments:
- Single-line comments start with `//`.
- Multi-line comments are enclosed between `/` and `/`.
|
|