|
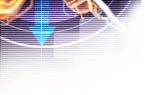 |
Ray-Tracing Vulkan API
Going Beyond Rasterization... |
|
|
|
 | Adding Feedback Loop to your Ray-Tracer (Path-Tracer) |  |
Path-tracers take many frames to converge on a final image. How do you take the output from the ray-tracer, store it and pass it to the next frames output?
You start with a ray-tracer which renders the output to the screen (single frame) - with no feedback, here is an example code:
Link for path-tracer code with no feedback (code).
The output for the ray-tracer is stored in a compute shader image (image2D ) so we'll use the same format for the stored texture that we'll pass to the next frame. The input from the last frame is the same as the one we write out.
|
|