|
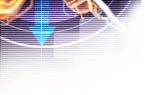 |
ShaderX..
Making our graphics card work for us.. |
|
|
|
 | Basic Effect Files with DirectX |  |
Vertex and Pixel shaders, and higher level languages such as Cg and HLSL can be combined into a single editable fx file, usually called Effect Files. The Effect Files can be opened in notepad and are basic text. We can set constants, variables, functions, define various techniques for different effects, even define which version of pixel or vertex shader it should be compiled to.
Effect files are a form of C language, slightly different though, but if you know C/C++ then it shouldn't be to hard to follow the codes workings.
File: basic.fx
|
|